Code Smell: Primitive Obsession
We coders can't even keep track of a few primitives. Can we just accept that and adjust our style or do we want to continue obsessing over primitive obsession?
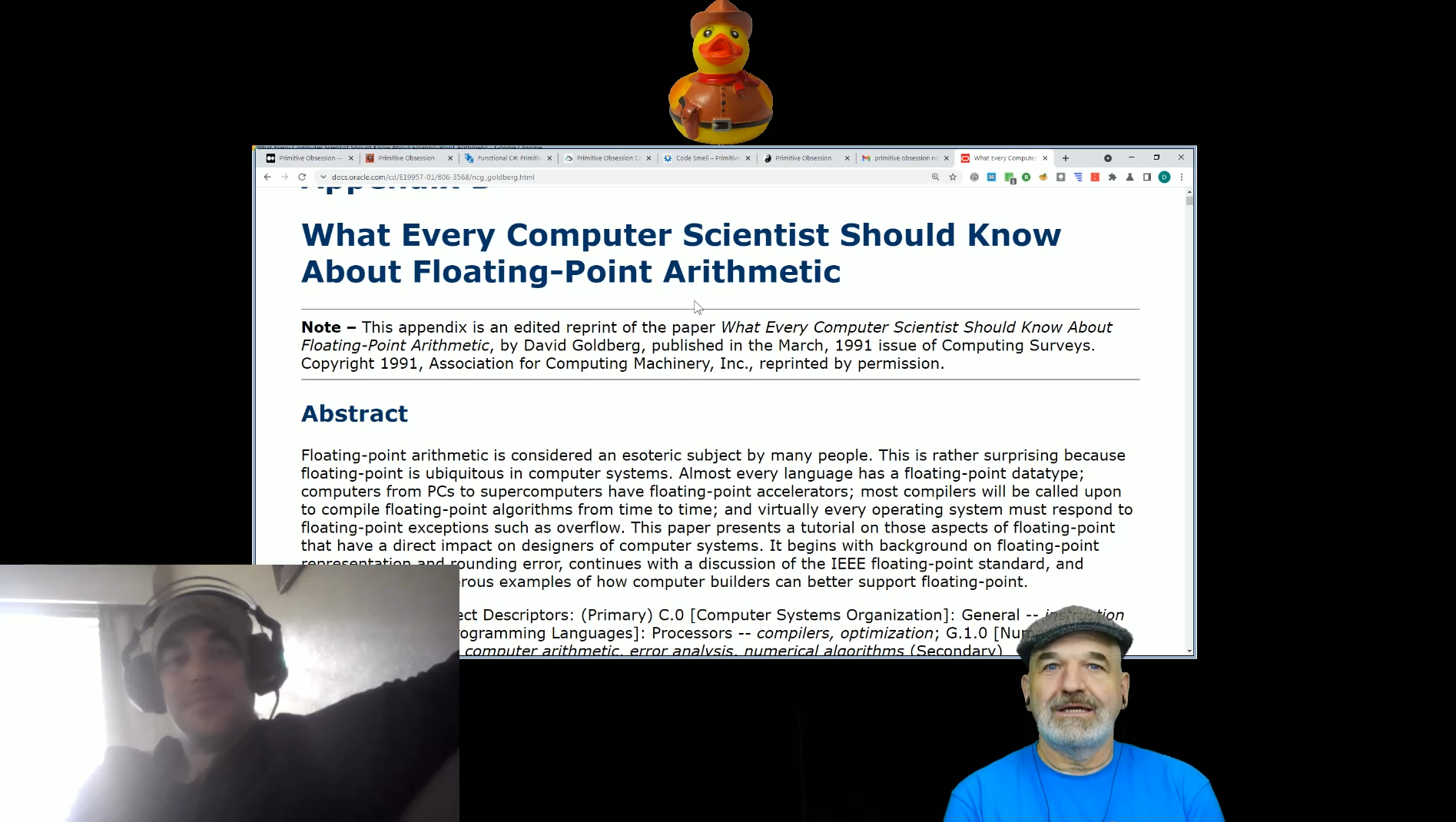
Technical Essays Referenced
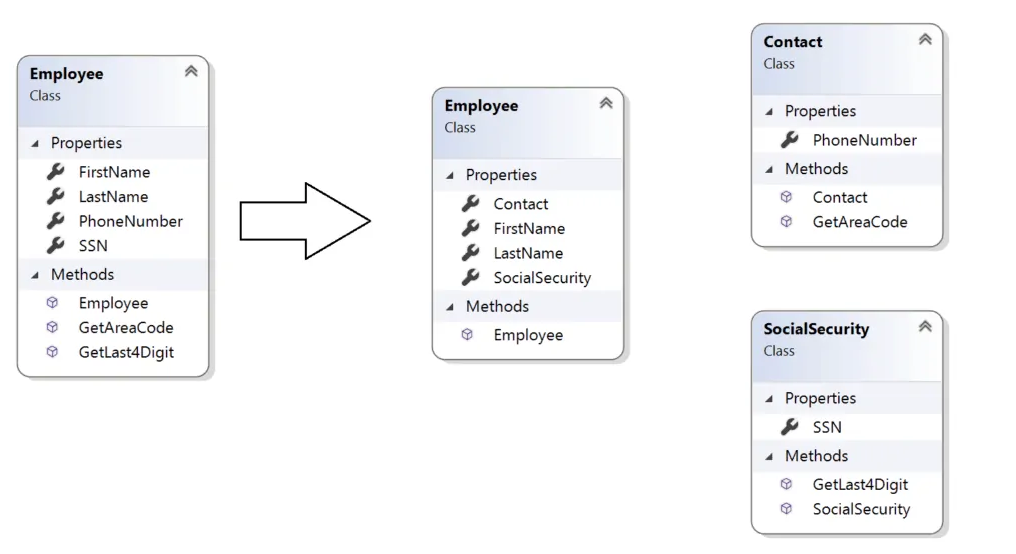
- Primitive Obsession — A Code Smell that Hurts People the Most
- Primitive Obsession
- Primitive Obsession Code Smell Resolution with example
- Code Smell
- Primitive Obsession
public class Customer
{
public string Name { get; private set; }
public string Email { get; private set; }
public Customer(string name, string email)
{
// Validate name
if (string.IsNullOrWhiteSpace(name) || name.Length > 50)
throw new ArgumentException("Name is invalid");
// Validate e-mail
if (string.IsNullOrWhiteSpace(email) || email.Length > 100)
throw new ArgumentException("E-mail is invalid");
if (!Regex.IsMatch(email, @"^([\w\.\-]+)@([\w\-]+)((\.(\w){2,3})+)$"))
throw new ArgumentException("E-mail is invalid");
Name = name;
Email = email;
}
public void ChangeName(string name)
{
// Validate name
if (string.IsNullOrWhiteSpace(name) || name.Length > 50)
throw new ArgumentException("Name is invalid");
Name = name;
}
public void ChangeEmail(string email)
{
// Validate e-mail
if (string.IsNullOrWhiteSpace(email) || email.Length > 100)
throw new ArgumentException("E-mail is invalid");
if (!Regex.IsMatch(email, @"^([\w\.\-]+)@([\w\-]+)((\.(\w){2,3})+)$"))
throw new ArgumentException("E-mail is invalid");
Email = email;
}
}
(Also of note, from Lobsters reader @corbin, "How Futile are Mindless Assessments of Roundoff in Floating-Point Computation?")
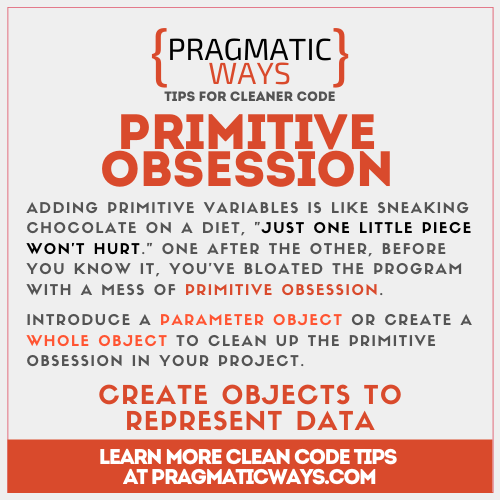
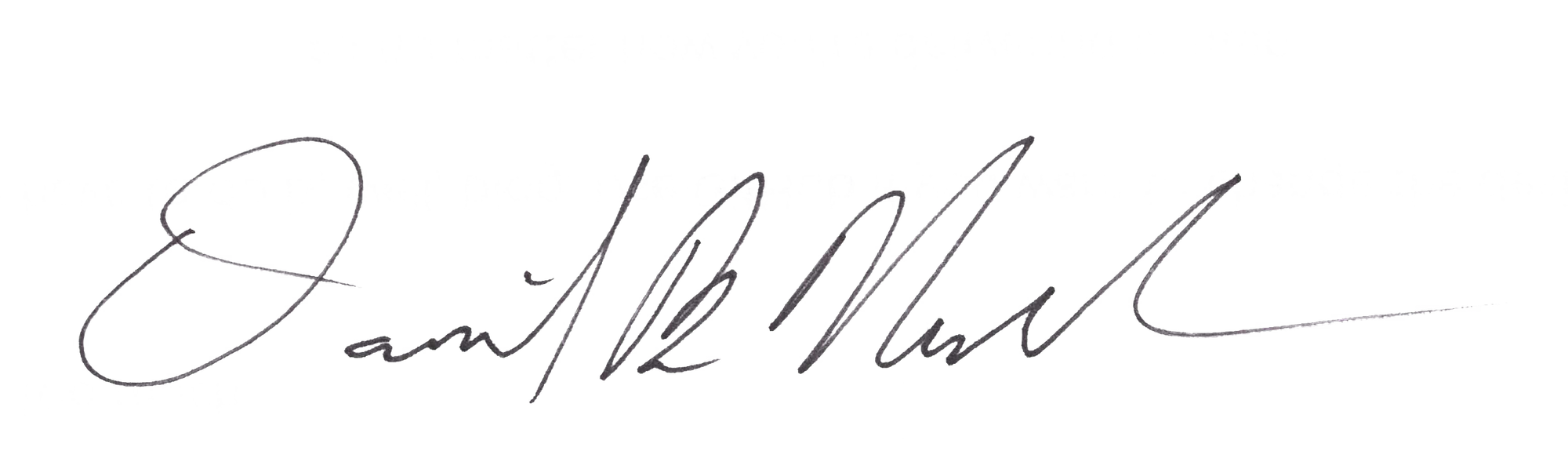
Comments ()